In this tutorial, we explore an innovative and practical application of IBM’s open-source ResNet-50 deep learning model, showcasing its capability to classify satellite imagery for disaster management rapidly. Leveraging pretrained convolutional neural networks (CNNs), this approach empowers users to swiftly analyze satellite images to identify and categorize disaster-affected areas, such as floods, wildfires, or earthquake damage. Using Google Colab, we’ll walk through a step-by-step process to easily set up the environment, preprocess images, perform inference, and interpret results.
First, we install essential libraries for PyTorch-based image processing and visualization tasks.
!pip install torch torchvision matplotlib pillow
We import necessary libraries and load the pretrained IBM-supported ResNet-50 model from PyTorch, preparing it for inference tasks.
import torch
import torchvision.models as models
import torchvision.transforms as transforms
from PIL import Image
import requests
from io import BytesIO
import matplotlib.pyplot as plt
model = models.resnet50(pretrained=True)
model.eval()
Now, we define the standard preprocessing pipeline for images, resizing and cropping them, converting them into tensors, and normalizing them to match ResNet-50’s input requirements.
preprocess = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(
mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225]
)
])
Here, we retrieve a satellite image from a given URL, preprocess it, classify it using the pretrained ResNet-50 model, and visualize the image with its top prediction. It also prints the top five predictions with associated probabilities.
def classify_satellite_image(url):
response = requests.get(url)
img = Image.open(BytesIO(response.content)).convert('RGB')
input_tensor = preprocess(img)
input_batch = input_tensor.unsqueeze(0)
with torch.no_grad():
output = model(input_batch)
labels_url = "https://raw.githubusercontent.com/pytorch/hub/master/imagenet_classes.txt"
labels = requests.get(labels_url).text.split("n")
probabilities = torch.nn.functional.softmax(output[0], dim=0)
top5_prob, top5_catid = torch.topk(probabilities, 5)
plt.imshow(img)
plt.axis('off')
plt.title("Top Prediction: {}".format(labels[top5_catid[0]]))
plt.show()
print("Top 5 Predictions:")
for i in range(top5_prob.size(0)):
print(labels[top5_catid[i]], top5_prob[i].item())
Finally, we download a wildfire-related satellite image, classify it using the pretrained ResNet-50 model, and visually display it along with its top five predictions.
image_url = "https://upload.wikimedia.org/wikipedia/commons/0/05/Burnout_ops_on_Mangum_Fire_McCall_Smokejumpers.jpg"
classify_satellite_image(image_url)
In conclusion, we’ve successfully harnessed IBM’s open-source ResNet-50 model in Google Colab to efficiently classify satellite imagery, supporting critical disaster assessment and response tasks. The approach outlined demonstrates the practicality and accessibility of advanced machine learning models and emphasizes how pretrained CNNs can be creatively applied to real-world challenges. With minimal setup, we now have a powerful tool at our disposal.
Here is the Colab Notebook. Also, don’t forget to follow us on Twitter and join our Telegram Channel and LinkedIn Group. Don’t Forget to join our 85k+ ML SubReddit.
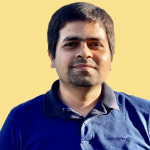
Asif Razzaq is the CEO of Marktechpost Media Inc.. As a visionary entrepreneur and engineer, Asif is committed to harnessing the potential of Artificial Intelligence for social good. His most recent endeavor is the launch of an Artificial Intelligence Media Platform, Marktechpost, which stands out for its in-depth coverage of machine learning and deep learning news that is both technically sound and easily understandable by a wide audience. The platform boasts of over 2 million monthly views, illustrating its popularity among audiences.